Here’s a step-by-step guide to help you prevent a 3D player character from falling over or rolling uncontrollably in Unity 3D. This issue is common in physics-based games, and addressing it ensures smoother player control, especially in games where the character should remain upright (such as first-person or third-person games).
Step 1: Set Up a RigidBody Component in Unity 3D
If you haven’t already, add a Rigidbody component to your player game object:
- Select the player object in the Hierarchy.
- In the Inspector, click on Add Component and search for Rigidbody.
- Adjust the Mass, Drag, and Angular Drag properties as needed. Setting a high Angular Drag can help reduce rotation over time.
Step 2: Lock Unwanted Rotations in Unity 3D
Unity’s Rigidbody component allows you to constrain specific axes of motion or rotation. This step helps prevent the player from rotating in unwanted directions:
- Go to the Rigidbody component in the Inspector.
- In the Constraints section, tick Freeze Rotation on the X and Z axes. This will prevent the Rigidbody from tilting forward, backward, or sideways.
- Leave the Y axis unchecked if you want the player to be able to turn horizontally. This is usually useful in games where the character can rotate around the Y-axis.
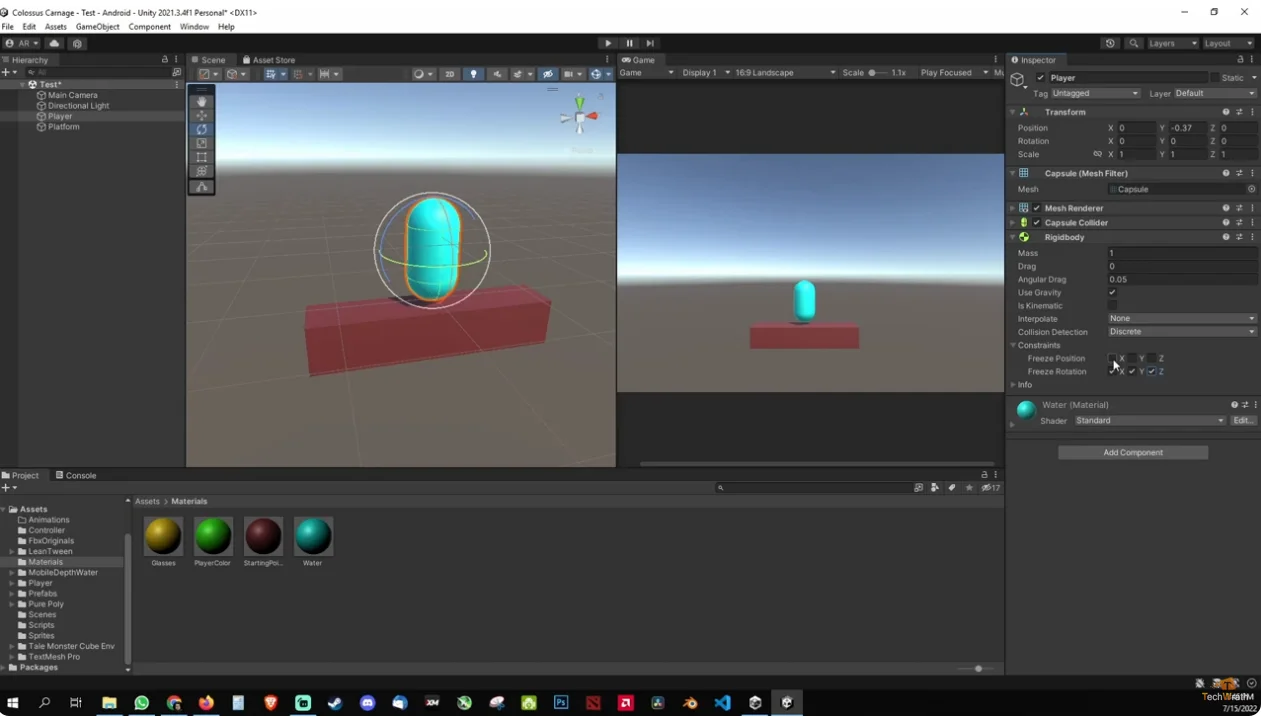
Step 3: Adjust the Collider Settings
Ensure that the Collider attached to your player is properly set up. If your Collider is off-center or doesn’t match the shape of your character, it could cause unexpected rotations:
- In the Inspector, select the Collider component attached to your player (e.g., Capsule Collider).
- Adjust the Center and Radius/Height values so the Collider encapsulates your character model accurately.
- If the character’s Collider is too wide or tall, the character may roll or tilt during movement.
Step 4: Use a Script to Maintain Upright Orientation
To ensure that the player remains upright at all times, you can apply a script that adjusts the player’s rotation every frame. This approach is especially useful if you need fine control over the player’s orientation in response to gameplay elements.
- Create a new C# script, for example, named StayUpright.cs.
- Open the script and add the following code:
using UnityEngine;
public class StayUpright : MonoBehaviour
{
public float rotationSpeed = 5f;
void FixedUpdate()
{
// Calculate target rotation
Quaternion targetRotation = Quaternion.Euler(0, transform.rotation.eulerAngles.y, 0);
// Smoothly rotate towards the target rotation
transform.rotation = Quaternion.Slerp(transform.rotation, targetRotation, rotationSpeed * Time.deltaTime);
}
}
- Attach this StayUpright script to your player object.
- Adjust the Rotation Speed variable in the Inspector. A higher value will keep the player more rigidly upright.
- Step 5: Test and Refine
- Play the scene and test how the player reacts to different movements and interactions.
- If the player is too rigid or has difficulty moving smoothly, lower the Rotation Speed or make adjustments to the Rigidbody and Collider settings.
- Optional: Adjust the Center of Mass
- You can adjust the center of mass to make the Rigidbody more stable:
- In the Inspector, add a Rigidbody property override.
- Set the center of mass lower on the Y-axis (e.g., -0.5 or lower) to give the Rigidbody a more grounded effect.
- Summary
- Add Rigidbody to the player object and adjust Angular Drag.
- Freeze X and Z rotation under Constraints in the Rigidbody component.
- Adjust the Collider to match the player’s shape.
- Attach StayUpright script to maintain an upright position.
- Optionally adjust the center of mass to make the Rigidbody more stable.
By following these steps, you’ll achieve a stable, upright player character in Unity that avoids unwanted rolling, tilting, or toppling, especially during sudden movements or physics interactions. This setup enhances player control, giving a more polished feel to gameplay, which is essential for player engagement and immersion.
Stabilizing your player character also helps you avoid bugs and inconsistencies in the game’s physics that can disrupt user experience. When your character remains upright and responsive, it conveys professional game design and smooth mechanics, making gameplay more enjoyable and accessible.
Unity offers endless possibilities for customizing player behavior, and fine-tuning such controls is a big step toward creating a successful game. With a solid grasp of Rigidbody settings, constraints, and orientation control, you can now explore advanced character controller scripts, add animations, or build more complex environments with confidence that your player will respond reliably.
Elevate Your Game with TechWrath Game Development Services
At TechWrath, we specialize in developing immersive, stable, and engaging gaming experiences that players love. From fine-tuning game physics to designing complex characters and environments, our team can bring your vision to life with expertise in Unity, Unreal Engine, and more. If you need guidance in building your next game or professional support for advanced development, TechWrath’s game development services are here to help you make a lasting impact. Contact us today to take your game to the next level!